Python class decorator tutorial
A Beginner’s Python Tutorial/Classes. From Wikibooks, open books for an open world < A Beginner's Python Tutorial. That is what functions cover in Python.
We could, of course, introduce a new decorator bunch_from_class() In Python the class statement creates the class body from the code you have written,
Class Based Decorators. If you want to maintain some sort of state and/or just make your code more confusing, you can also use class based decorators.
In the snippet shown above, we decorate the class method print_test. The method_decorator prints the name of the city, if the name of city instance is not SFO.
This is exactly what the decorators do in Python! They wrap a function and modify its behaviour in one way or the another. 7.6.2. Decorator Classes
Python 2.7 This tutorial deals with Python Version 2.7 Class decorators A decorator in Python is any callable Python object that is used to modify a function or a
Another option is to use a built-in decorator from Python that would allow you to do exactly what Decorating classes is a bit of a In this tutorial,
LearnPython.org is a free interactive Python tutorial for people who want to learn Python, Classes and Objects. A very basic class would look something like this:
Let's start with a function in Python. To understand decorators, we need to know the full scope of capabilities of Python functions. Everything in Python is an object.
Quick Tutorial: Introduction to Python Decorators. First, in Python a function is an (first-class) A decorator function in Python usually has at least one
Python Decorator Example, Decorator Python, Python Decorators, python method decorator, python decorator with function arguments, exception handling.
A complete Python Decorators Tutorial that explains what are decorators in Python, and how to create and use them to write better and cleaner code. A decoratr is a
Decorators with Arguments. Now let’s modify the above example to see what happens when we add arguments to the decorator: class decoratorWithArguments(object): def
Today post will be about syntactic sugar of python language-decorators.I will concentrate on class decorators. Let’s start with basic example of decorator defined
Python Built-in Decorators
Python2 Tutorial Easy Introduction into Decorators and
When function decorators were originally debated for inclusion in Python 2.4, class decorators were seen as obscure and unnecessary [1] thanks to metaclasses. After
Serializable decorator for Python Class 08 Jan 2010. I am going to describe a quick recipe for adding serializability to a Python Class. I was playing with Redis
Python Decorators Overview. (That’s what it means when people say Python has first class functions.) Decorators are a concise way of taking A Tutorial and
Are decorators getting in the way? In this tutorial, you’ll gain a deeper understanding of Python decorators.
Decorators have something in common with previous metaprogramming abstractions introduced to Python: they do not actually do anything you could not do without them
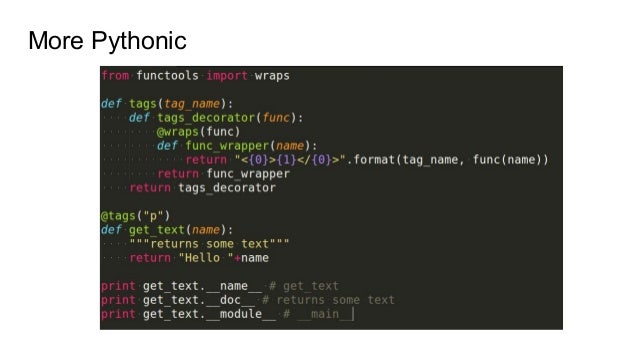
4/04/2016 · In this Python tutorial, we will be learning about decorators. Decorators are a way to dynamically alter the functionality of your functions. So for
You will learn about Python @property; Python Tutorial. Python Introduction. Python Object & Class. Python OOP; Python Class;
Python Class Decorator. Ask Question. Browse other questions tagged python decorator or ask your own question. asked. 8 years ago. viewed. 2,563 times

In this tutorial, learn how to implement decorators in Python.
Indeed, you can use Python decorators to implement the Decorator pattern, but that’s an extremely limited use of it. and in the case of class decorators,
The @ classmethod decorator is used to create singleton classes. This is a Python technique for defining an object which is also a unique class.
Chapter 25 – Decorators¶ Python decorators are really cool, but they can be a little hard to understand at first. A decorator in Python is a function that accepts
Python: All About Decorators. added to make decorating functions and methods possible in Python 2.4. Class decorators were proposed in PEP 3129 to Tutorial
Latest Python Tutorials: Python Decorators: A Step-By-Step Introduction. moment to refresh your memory on the properties of first-class functions in Python.
In Python 2.5, is there a way to create a decorator that decorates a class? Specifically, I want to use a decorator to add a member to a class and change the
The functools module is for this class decorator supplies It is made available in this module to allow writing code more forward-compatible with Python 3.
Decorators With Arguments in Python – Scott Lobdell
(16 replies) Hi, I am learning python by learning django, and I stumble upon decorator which is very cool, any beginners resources for python decorators, although I
19/08/2016 · In this Python Object-Oriented Tutorial, we will be learning about the property decorator. The property decorator allows us to define Class methods that we
LearnPython.org is a free interactive Python tutorial for people who want to learn Python, Classes and Objects; Decorators; Other Python Tutorials.
A very simple code example python tutorial decorators function in introduction learn 30sec microsoft awarded mvp python decorator Python Class Decorator Tutorial;
comprehensive python decorator tutorial In the snippet shown above, we decorate the class method print_test. The method_decorator prints the name of the city, if
This tutorial explores metaprogramming in Python. You can see it as having a class decorator with the difference that __init__ would be
Decorator functions are software design patterns. They dynamically alter the functionality of a function, method, or class without having to directly use subclasses
Python Higher-Order Functions and Decorators. In Python, functions are treated as first class objects, allowing you to perform the following operations on functions.
In this article, you will learn about Python decorators, the underlying mechanism behind decorators and how are decorators created in Python.
Python Design Patterns Decorator – Learn Python Design Patterns in simple and easy steps starting from basic to advanced concepts with examples including Introduction – 18th century interior design materiality pdf This tutorial helps demystify what’s behind class, static, and instance methods in Python decorator to flag it as a static tutorial at Real Python is created
Getting Started. Decorators are a pretty cool concept that allow you to decorate functions and classes with a decorator and a new, transformed function or class will
This post gives examples of 3 different ways to write Python decorators, and it talks further about catching extra function arguments, callable classes, and the
What is Decorator? Python “decorator” is a language construct that lets you define a wrapper function g to another function f, such that, when f is called, your
This tutorial aims to introduce more interesting uses of Python decorators, specifically how decorators can be used on classes and how to pass extra parameters to
Python property decorator, python @property, Python property example, Python property setter, property python, python class property decorator setter code
What are decorators in Python? everything in Python (Yes! Even classes), Python Tutorial. Python Introduction. Getting Started;
Write Your Own Python Decorators Code Envato Tuts+
In this post I will be discussing Python’s function decorators in depth, In Python, functions are first class citizens, Python’s Decorator Syntax.
Python 3 This is a tutorial in Python3, Class decorators A decorator in Python is any callable Python object that is used to modify a function or a class.
Decorator inside Python class. Let’s suppose we need a decorator that we gonna use inside one specific class only. Something like this:
Class decorators before Python 2.6 Prior to Python 2.6 one could not write @a_decorator classMyClass Objects and classes in Python Documentation,
In Python, a function is a first-class Concluding what we discussed so far in this Python Decorator with arguments simple examples Tutorial, decorators in python
Decorators for Functions and Methods: Author: PEP 3129 [#PEP-3129] proposes to add class decorators as of Python 2.6. Why Is This So Hard? Two decorators
Python Programming tutorials from beginner to advanced on a massive variety of from our new parent class, add_wrapping_with Decorators in Python Tutorial.
Improve Your Python: Python Classes and Object Oriented Programming. Jeff Knupp PYTHON PROGRAMMER it is passed the class. It, too, is defined using a decorator:
Decorators in Python Tutorial Python Programming Tutorials

Python class @decorators Krzysztof Żuraw Blog
As a Python instructor, understanding decorators is a topic I Functions are first class objects in Python. Be sure to read the Python Tutorial for more
Decorators¶ This section cover the decorator syntax and the concept of a decorator (or decorating) callable. Decorators are a syntactic convenience, that allows a
19/12/2016 · In this tutorial, I explain decorators in a very simple way by going over how to measure execution time of function using decorators. They serve as a
In this introductory tutorial, we’ll look at what Python decorators are and how to create and use them. all the decorators you saw above will work as class
Python Decorators How to Use it and Why? Programiz

Decorators python Tutorial
The @ is a special syntax that applies the decorator function to the underneath function or class. Here, cache(func) is called, passing original func
After trying for about the fifth time, I think I am starting to understand Python decorators due largely to Jack Diederich’s PyCon 2009 talk, Class Decorators
Write Your Own Python Decorators In this tutorial I’ll show you how to write Class decorators in Python 3 add a whole new dimension by customizing the
Decorators make magic easy – IBM Developer
Python decorator tutorial Ha.Minh’s Blog – GitHub Pages
Two of the simplest Python decorator examples SaltyCrane
– Chapter 25 Decorators — Python 101 1.0 documentation
Python Decorators Tutorial TutorialEdge.net
[Python] decorators tutorials Grokbase
Decorators — Objects and classes in Python tutorial
Python Class Decorator Stack Overflow
Python Built-in Decorators
In the snippet shown above, we decorate the class method print_test. The method_decorator prints the name of the city, if the name of city instance is not SFO.
LearnPython.org is a free interactive Python tutorial for people who want to learn Python, Classes and Objects; Decorators; Other Python Tutorials.
In this post I will be discussing Python’s function decorators in depth, In Python, functions are first class citizens, Python’s Decorator Syntax.
LearnPython.org is a free interactive Python tutorial for people who want to learn Python, Classes and Objects. A very basic class would look something like this:
Decorators have something in common with previous metaprogramming abstractions introduced to Python: they do not actually do anything you could not do without them
Decorators with Arguments. Now let’s modify the above example to see what happens when we add arguments to the decorator: class decoratorWithArguments(object): def
The @ classmethod decorator is used to create singleton classes. This is a Python technique for defining an object which is also a unique class.
Another option is to use a built-in decorator from Python that would allow you to do exactly what Decorating classes is a bit of a In this tutorial,
When function decorators were originally debated for inclusion in Python 2.4, class decorators were seen as obscure and unnecessary [1] thanks to metaclasses. After
Today post will be about syntactic sugar of python language-decorators.I will concentrate on class decorators. Let’s start with basic example of decorator defined
Python Class Decorator. Ask Question. Browse other questions tagged python decorator or ask your own question. asked. 8 years ago. viewed. 2,563 times
In this tutorial, learn how to implement decorators in Python.
This tutorial explores metaprogramming in Python. You can see it as having a class decorator with the difference that __init__ would be
Python2 Tutorial Easy Introduction into Decorators and
Decorators python Tutorial
Python Design Patterns Decorator tutorialspoint.com
Python Programming tutorials from beginner to advanced on a massive variety of from our new parent class, add_wrapping_with Decorators in Python Tutorial.
Python Decorator Example JournalDev
[Python] decorators tutorials Grokbase